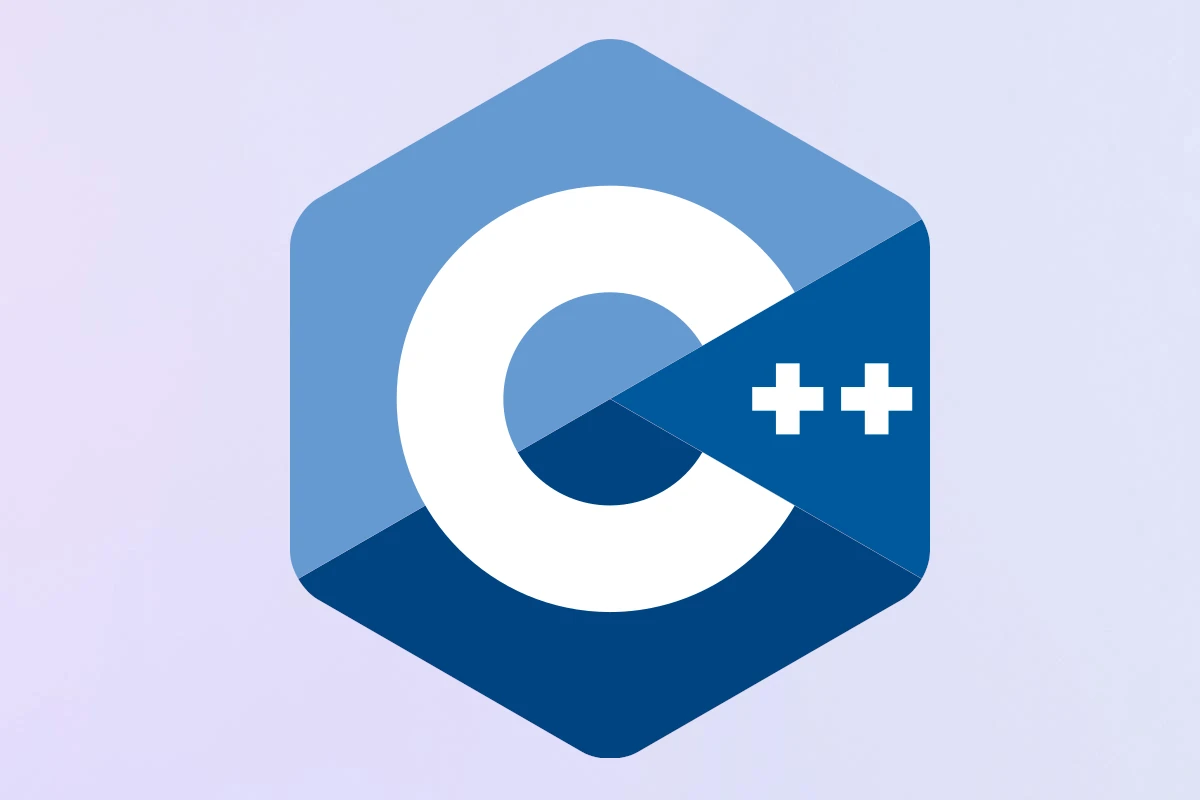
Converting a string to an int in C++ is a common task that many programmers need to perform. Whether you’re dealing with user input or processing data, knowing how to handle these conversions efficiently can save a lot of time. The most straightforward way to convert a string to an int in C++ is by using the std::stoi
function.
Several methods exist for converting strings to integers in C++, each with its advantages. Besides std::stoi
, techniques like using the stringstream
class offer flexibility and simplicity. Beginners and experienced programmers alike can benefit from understanding these different approaches to choose the best method for their specific needs.
Key Takeaways
- String-to-int conversion in C++ can be done using
std::stoi
andstringstream
. - These methods help handle user inputs and data processing effectively.
- Choosing the right conversion method ensures efficient and error-free code.
Understanding C++ String and Int Types
When working with C++ programming, it’s essential to understand the std::string
class and the integer data types. These two types are fundamental in managing textual and numerical data effectively.
The std::string Class
C++ provides a std::string
class to handle strings. This class includes many methods to manipulate text data. For instance, std::string::size()
returns the number of characters in the string, while std::string::empty()
checks if it contains any characters.
To add characters, methods like insert()
, append()
, and push_back()
are available. You can search for specific characters or substrings using find()
, find_first_not_of()
, and find_last_not_of()
. Furthermore, extracting a part of a string can be done with substr()
. Comparing strings is straightforward with the compare()
function.
C++11 introduced the std::to_string()
method to convert numbers to their string representation. Moreover, std::string
also supports operations like operator+
for concatenation and assign()
to replace content. Key members like data()
, c_str()
, begin()
, end()
, front()
, and back()
provide direct access to underlying character data.
The Integer Data Type in C++
The integer data type in C++ is used for storing whole numbers. They come in various sizes: int
, short
, long
, and long long
. Converting a string to an integer is a common task in C++. The stoi()
function is a simple way to convert a string to an int
.
Another option is using std::stringstream
from the <sstream>
header. This class lets you treat a string as a stream, making it easy to extract integers from the text. The atoi()
function converts a C-style string (const char*
) to an integer. However, this function might be less safe than std::stoi()
because it doesn’t handle errors as robustly.
Understanding these data types and their functions can help manage and manipulate textual and numerical data efficiently in C++. This knowledge enables precise and efficient programming in various applications.
Methods of Converting String to Int in C++
Converting strings to integers in C++ can be done in different ways depending on the requirements and the version of C++ being used. This section explores three main methods: using stoi()
, stream-based conversions, and other alternative techniques.
Using stoi() and Related Functions
C++11 introduced the std::stoi()
function, which is a straightforward way to convert a string to an int. It can handle leading whitespace and stops conversion at the first non-numeric character. To use this function, include the <string>
header file.
Example:
std::string str = "12345";
int num = std::stoi(str);
If the conversion fails, std::stoi()
will throw std::invalid_argument
if no conversion could be performed, or std::out_of_range
if the converted value is out of the range of representable values. Additionally, there are related functions such as std::stol()
and std::stoll()
for converting to long and long long, respectively.
Stream-Based Conversions with stringstream
Another common method involves using the stringstream
class from the <sstream>
header. It treats strings like a stream of data and can easily convert a string to an int.
Example:
#include <sstream>
std::string str = "12345";
std::stringstream ss(str);
int num;
ss >> num;
This method can be useful when working with mixed data types because the stream can be reused for different conversions. However, it may be less efficient compared to std::stoi()
due to the overhead of stream operations.
Alternative Conversion Techniques
Other methods include the use of functions like atoi()
from the <cstdlib>
header, although it’s considered less safe since it doesn’t handle errors well.
Example:
#include <cstdlib>
std::string str = "12345";
int num = std::atoi(str.c_str());
Additionally, sscanf()
can be useful for more complex parsing needs, though it requires greater attention to detail.
Example:
#include <cstdio>
std::string str = "12345";
int num;
sscanf(str.c_str(), "%d", &num);
For those using Boost libraries, boost::lexical_cast
can be an elegant solution. It offers simpler syntax while still maintaining error handling capabilities.
Example:
#include <boost/lexical_cast.hpp>
std::string str = "12345";
int num = boost::lexical_cast<int>(str);
These alternative methods provide flexibility depending on the specific needs of the application and the environment.
Frequently Asked Questions
These FAQs address common ways to convert strings to various numeric types in C++. Methods for converting strings to integers, doubles, and other formats are covered.
How can I convert a string to an integer in C++ without using stoi?
To convert a string to an integer without stoi
, use the stringstream
class in the <sstream>
header. Initialize a stringstream
with the string, then extract the integer. Example:
#include <sstream>
#include <string>
#include <iostream>
int stringToInt(const std::string &str) {
std::stringstream ss(str);
int number;
ss >> number;
return number;
}
What is the appropriate method to convert a string to a double in C++?
Use std::stod
from the C++ Standard Library to convert a string to a double. Example:
#include <string>
#include <iostream>
int main() {
std::string str = "123.45";
double num = std::stod(str);
std::cout << num;
return 0;
}
Is there a standard way to convert characters to integers in C++?
Yes, use the atoi
function from the C Standard Library to convert a string representing digits to an integer. Ensure the string is valid. Example:
#include <cstdlib> // for atoi
#include <iostream>
int main() {
const char* str = "456";
int num = std::atoi(str);
std::cout << num;
return 0;
}
How do you transform a C++ string into a float type?
Use std::stof
to convert a string to a float. Example:
#include <string>
#include <iostream>
int main() {
std::string str = "123.456";
float num = std::stof(str);
std::cout << num;
return 0;
}
What is the process for converting a string to an int64_t in C?
Use the strtoll
function to convert a string to an int64_t
. Example:
#include <cstdlib> // for strtoll
#include <iostream>
int main() {
const char* str = "9223372036854775807";
int64_t num = std::strtoll(str, nullptr, 10);
std::cout << num;
return 0;
}
How can a string be converted to a long long integer in C++?
Use std::stoll
to convert a string to a long long int
. Example:
#include <string>
#include <iostream>
int main() {
std::string str = "9876543210";
long long int num = std::stoll(str);
std::cout << num;
return 0;
}