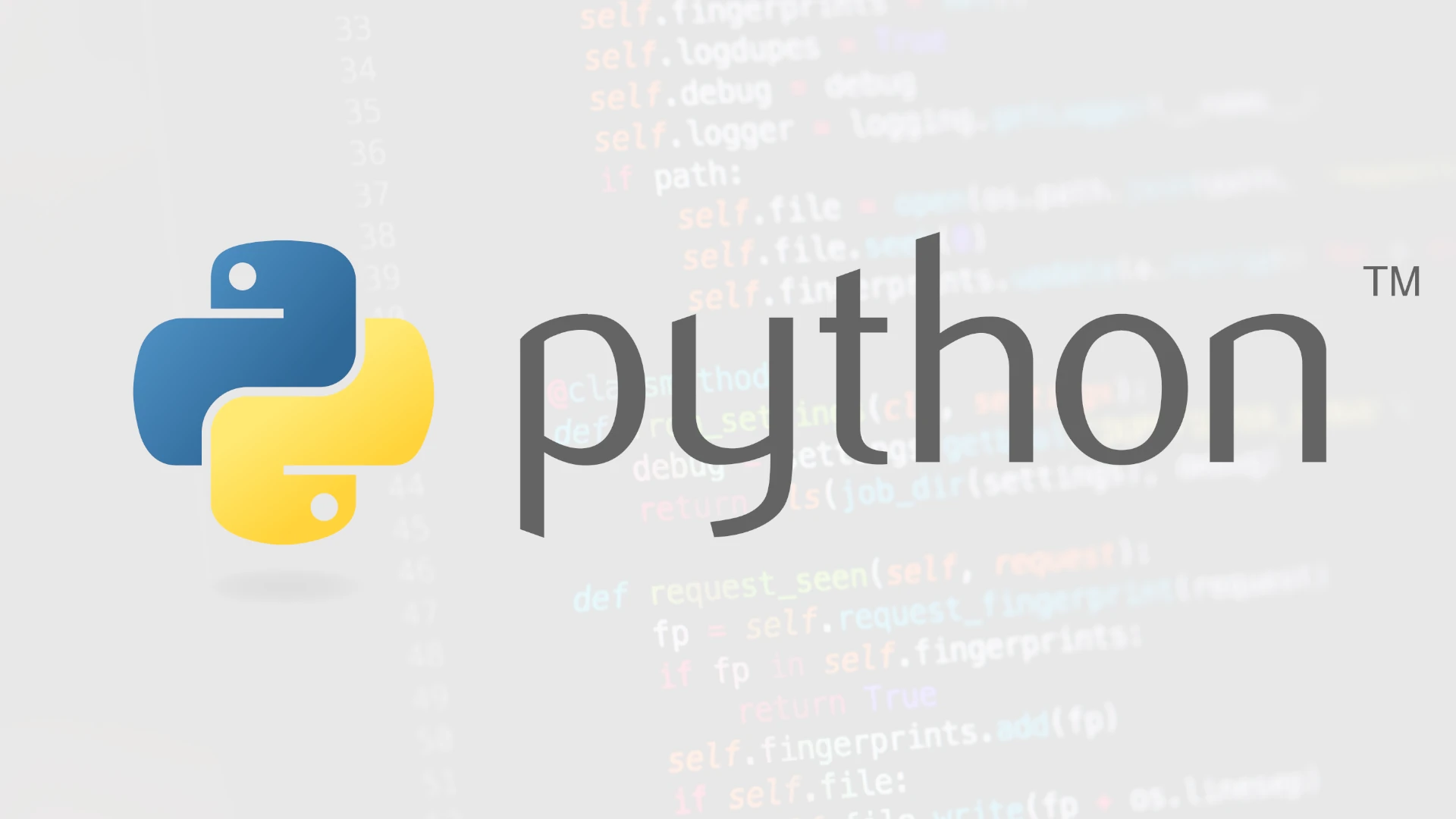
F-strings in Python make string formatting much simpler and more intuitive. Introduced in Python 3.6, f-strings allow you to embed expressions directly within string literals. By placing an ‘f’ or ‘F’ before the opening quote, you can include variable names inside curly braces, which will then be replaced with their values.
This method is both faster and more readable compared to older formatting techniques like the % operator or the str.format() method. For instance, instead of writing 'Hello, {}'.format(name)
, you can simply write f'Hello, {name}'
.
Streamlining String Formatting with Python’s f-strings
Concise Syntax for Embedded Expressions
Python’s f-strings, introduced in Python 3.6, provide a concise way to embed expressions directly within string literals. By prefixing a string with the letter “f” or “F”, you unlock the ability to insert variables, expressions, and even function calls seamlessly into your strings.
Enhanced Readability
f-strings prioritize readability by allowing you to place expressions where they logically belong, directly within the string itself. This eliminates the need for complex formatting placeholders or additional function calls, making your code cleaner and easier to understand.
Dynamic String Generation
With f-strings, you can dynamically generate strings based on the values of variables or expressions. This makes it ideal for tasks like logging messages, building formatted reports, or creating user-friendly output.
Formatting Options Galore
f-strings support a wide range of formatting options, including specifying the number of decimal places for floats, aligning text, padding values, and even converting numbers to different bases. This flexibility empowers you to create precisely formatted output with minimal effort.
Examples of f-strings in Action
name = "Alice"
age = 30
greeting = f"Hello, {name}! You are {age} years old."
print(greeting) # Output: Hello, Alice! You are 30 years old.
price = 19.99
formatted_price = f"The price is ${price:.2f}"
print(formatted_price) # Output: The price is $19.99
numbers = [1, 2, 3]
string_of_numbers = f"Numbers: {', '.join(map(str, numbers))}"
print(string_of_numbers) # Output: Numbers: 1, 2, 3
Key Advantages of Python f-strings
Feature | Description |
---|---|
Concise Syntax | f-strings offer a streamlined syntax for embedding expressions within strings. |
Improved Readability | Expressions are placed where they logically belong, enhancing code clarity. |
Dynamic Formatting | Easily generate strings based on variable values or expressions. |
Versatile Formatting Options | Control the presentation of numbers, text, and more with a wide range of formatting options. |
Performance Boost | f-strings are often faster than older string formatting methods like % formatting or .format() . |
Key Takeaways
- F-strings simplify string formatting
- Introduced in Python 3.6, they embed expressions directly
- They are faster and more readable than older techniques
Understanding Python F-Strings
Python f-strings make it easy to format strings. Introduced in Python 3.6, they provide a more straightforward way to embed variables and expressions directly in the string.
Historical Context: Python 3.6 and PEP 498
Python f-strings were introduced with Python 3.6 in PEP 498. Before f-strings, Python used %
and str.format()
for string formatting. Both methods required more code and were less readable. PEP 498 proposed f-strings, or formatted string literals, to make strings easier to read and write.
By using curly braces {}
to embed variables and expressions, f-strings simplified string formatting. The syntax is both cleaner and faster, so f-strings quickly became the preferred method.
Basic Syntax of F-Strings
F-strings use a simple and clear syntax. Start an f-string with an f
or F
before the opening quote. Inside the string, use curly braces {}
to embed variables or expressions.
name = "Alice"
f_string = f"Hello, {name}!"
print(f_string) # Output: Hello, Alice!
This example shows how to include a variable directly in the string. You can also place expressions, making f-strings very flexible.
Embedding Expressions and Variables
F-strings allow you to embed any valid Python expression inside curly braces. This includes arithmetic operations, function calls, and method calls.
value = 10
f"Half of {value} is {value / 2}"
The embedded expression {value / 2}
evaluates at runtime. You can even call functions within f-strings.
def greet(name):
return f"Hello, {name}"
f"{greet('Bob')}"
This flexibility makes f-strings powerful for dynamic string creation.
Special Characters and Escaping Braces
Sometimes you need to include special characters or literal braces in an f-string. Use a backslash \
to escape braces {}
.
f"{{This is not a placeholder}}, but this is: {5 * 2}"
The output will be {This is not a placeholder}, but this is: 10
. To include special characters like newlines \n
or tabs \t
, you use them as you would in any Python string.
f"Line 1\nLine 2"
This shows the flexibility in handling spaces and special characters in formatted strings.
Debugging with F-Strings
F-strings help with debugging by allowing embedded variables and expressions with additional debugging details. Use the =
sign inside the curly braces to include both the variable name and its value.
value = 42
f"{value=}"
Output will be value=42
, making it easier to understand what’s happening in your code. This feature is especially useful when printing multiple variables for debugging purposes.
F-strings offer a clear and powerful way to format strings in Python. Their simplicity and flexibility help make Python code more readable.
Advanced Formatting with F-Strings
In Python, f-strings provide powerful tools for advanced string formatting, combining readability with efficiency. Below, we explore various techniques such as string interpolation, format specifiers, numeric formatting, and handling complex objects.
String Interpolation
String interpolation lets you insert values directly into strings using curly braces {}
. It simplifies combining text and variables. In f-string syntax, prefix your string with f
and place variables inside curly braces.
name = "Alice"
greeting = f"Hello, {name}!"
You can also include expressions:
age = 30
message = f"In ten years, {age + 10} years old."
The expressions inside the braces get evaluated at runtime, making this approach concise and readable.
Format Specifiers and Alignment
Format specifiers control how values appear. Using a colon :
inside curly braces allows fine-tuned formatting.
For example, to add padding or align text:
text = "test"
padded_text = f"{text:>10}" # Right-align within 10 spaces
Align options:
:<
for left-align:>
for right-align:^
for center-align
Specifiers also handle number formatting with precision:
number = 123.456
formatted_number = f"{number:.2f}" # Two decimal places
Using these specifiers enhances the presentation of your data, making it clear and organized.
Numeric and Date Formatting
F-strings allow easy number formatting. You can format integers in various bases, such as decimal, hexadecimal, or octal.
num = 255
formatted_num = f"{num:#x}" # Hexadecimal with prefix
Scientific notation and number separators are also possible:
large_num = 1000000
formatted_large_num = f"{large_num:,}" # 1,000,000
Date and time formatting is straightforward using the datetime
module.
from datetime import datetime
now = datetime.now()
formatted_date = f"{now:%Y-%m-%d %H:%M:%S}"
These formats ensure clarity and consistency in presenting dates and numbers.
Handling Complex Objects
F-strings handle dictionaries and objects.
For dictionaries:
data = {"key": "value"}
entry = f"Data: {data['key']}"
For class objects, you can customize their display with the __str__
or __repr__
methods.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"{self.name}, {self.age} years old"
person = Person("Bob", 25)
info = f"Person info: {person}"
Make sure your objects include meaningful __repr__
or __str__
methods to take full advantage of f-strings. This removes any boilerplate code, providing a cleaner and more readable format.
Frequently Asked Questions
Python’s f-strings are a powerful tool for string formatting. They make code more readable and efficient by embedding expressions directly within string literals. Below are some common questions about using f-strings in Python.
How can you perform string formatting using f-strings in Python?
F-strings use curly braces {}
to include expressions inside string literals. Start the string with an f
or F
before the opening quotation mark. For example, name = "Alice"
, and f"Hello, {name}!"
results in “Hello, Alice!”.
What is the correct way to format a float to two decimal places using f-strings?
To format a float to two decimal places, use :.2f
inside the curly braces. For example, value = 3.14159
, and f"{value:.2f}"
results in “3.14”.
Can you provide an example of multiline f-string usage in Python?
Multiline f-strings are created using triple quotes. For example:
name = "Alice"
age = 30
details = f"""
Name: {name}
Age: {age}
"""
This will produce:
Name: Alice
Age: 30
How do you include curly braces within the text of an f-string?
To include curly braces, double them. For example, f"{{Hello}}"
would result in “{Hello}”. This way, the braces are treated as literal characters.
Are f-strings considered more efficient than the traditional .format method?
Yes, f-strings are generally faster than the .format
method. This is because f-strings are evaluated at runtime, and the expressions are converted to their values directly inside the string.
What does the format specifier ‘.2f’ represent in the context of f-strings?
The .2f
specifier formats a float to two decimal places. For example, with number = 3.14159
, using f"{number:.2f}"
results in “3.14”. This is useful for controlling the precision of floating-point numbers.