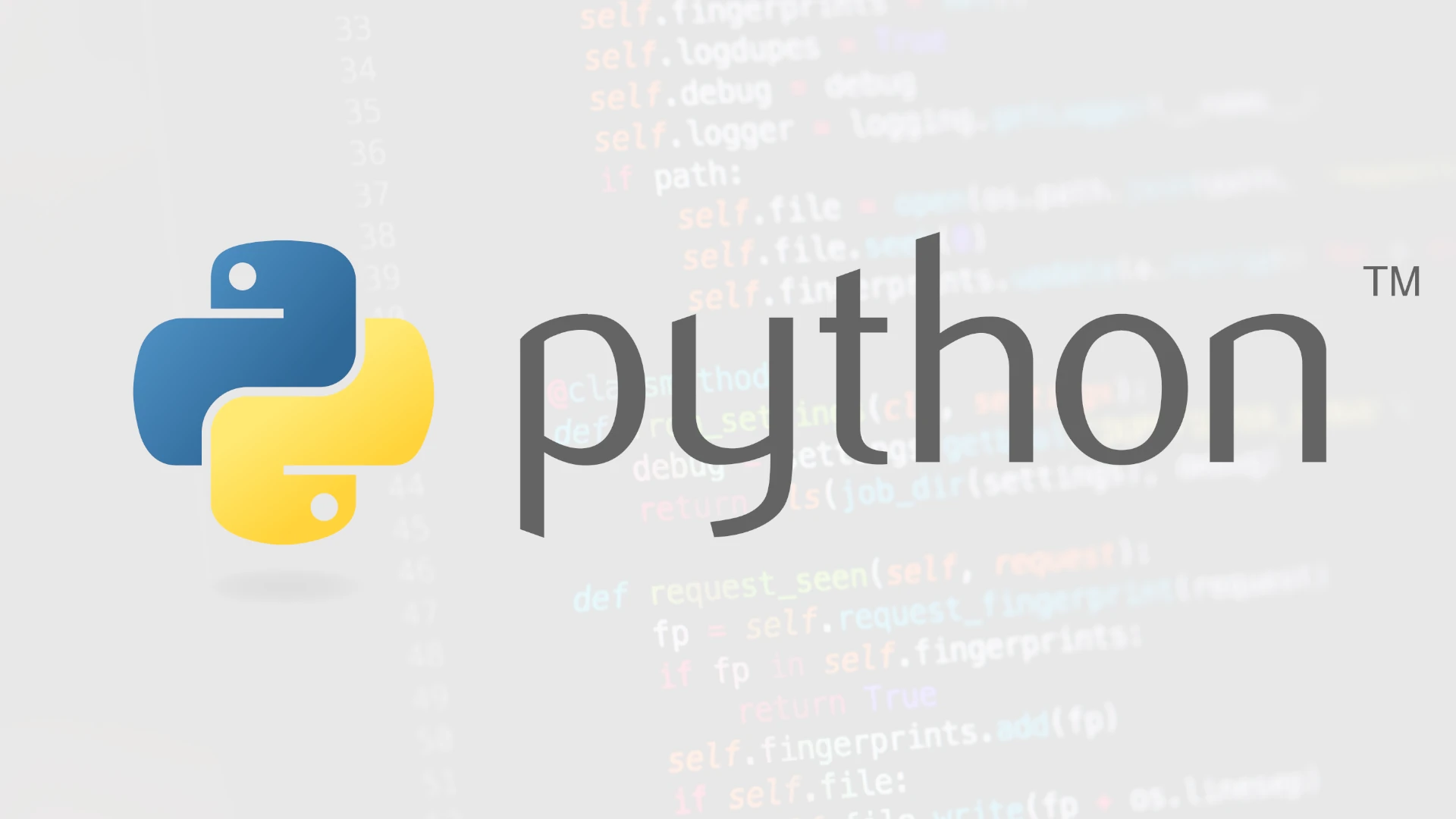
Reading a JSON file with Python is an essential skill for anyone working with web applications or data formats. Using Python’s built-in JSON module, you can quickly load and parse JSON data into a Python dictionary. This enables developers to easily manipulate and work with data in their programs.
The process involves reading JSON from a file and converting it into a format that Python can understand. You’ll use methods like json.load()
or json.loads()
to handle the data efficiently. This quick read ensures that your application processes information smoothly and accurately, aligning perfectly with your data needs.
Whether you are dealing with simple configurations or complex nested structures, Python’s JSON library makes the job straightforward. Understanding this technique will improve your ability to build more dynamic and responsive web applications.
Mastering JSON Data with Python
The json
Module: Your Go-To Tool
Python’s built-in json
module is the cornerstone of working with JSON data. It provides functions to seamlessly convert JSON strings into Python objects (dictionaries, lists, etc.) and vice-versa.
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
Reading from Strings: json.loads()
When your JSON data is already in a string format, json.loads()
comes to the rescue. It parses the string and returns the corresponding Python object.
json_string = '{"name": "Alice", "age": 30}'
data = json.loads(json_string)
print(data['name']) # Output: Alice
Handling Large Files: ijson
Library
For hefty JSON files, ijson
offers a memory-efficient solution. It processes the file incrementally, preventing your system from being overwhelmed by large datasets.
import ijson
with open('large_data.json', 'r') as file:
items = ijson.items(file, 'item')
for item in items:
print(item)
Streaming JSON Data: jsonlines
When dealing with newline-delimited JSON (NDJSON), jsonlines
simplifies the reading process. It handles each line as a separate JSON object, making it perfect for streaming data.
import jsonlines
with jsonlines.open('data.jsonl') as reader:
for obj in reader:
print(obj)
Key Considerations
- Error Handling: Always wrap your JSON operations in
try-except
blocks to catch potential errors likeJSONDecodeError
. - Data Validation: Ensure the JSON structure aligns with your expectations before processing it.
- Performance: For massive JSON files, consider libraries like
ijson
orjson-stream
for optimized performance.
Python JSON Libraries: A Quick Comparison
Library | Purpose | Benefits |
---|---|---|
json | Built-in, basic JSON handling | Simple, reliable, sufficient for most cases |
ijson | Parsing large JSON files | Memory efficient, ideal for big datasets |
jsonlines | Reading NDJSON files | Easy streaming of JSON objects |
json-stream | Parsing large JSON streams | Handles continuous JSON data efficiently |
Key Takeaways
- Python’s JSON module makes it easy to read and parse JSON files.
- Using
json.load()
helps convert JSON data into a Python dictionary. - Mastering these techniques improves data handling in web applications.
Reading JSON Files in Python
Python provides several tools for reading JSON files and converting these files into Python objects for easy data manipulation and usage in various applications.
Understanding the JSON Format
JSON, or JavaScript Object Notation, is a lightweight data interchange format. It uses human-readable text to store and transmit data objects consisting of attribute-value pairs. Key elements include:
- Objects: Enclosed in curly braces
{ }
, with key/value pairs. - Arrays: Collections of values enclosed in square brackets
[ ]
. - Values: Can be a string, number, object, array,
true
,false
, ornull
.
Example:
{
"name": "John",
"age": 30,
"isStudent": false,
"courses": ["Math", "Science"]
}
Setting Up the Python Environment
To handle JSON files, Python uses the built-in json
module. The following steps are necessary:
- Import the Module: Use
import json
. - Open the File: Use the built-in
open()
function to access the file. - Read the Data: Use methods provided by the
json
module to decode the data.
import json
with open('example.json') as file:
data = json.load(file)
Ensure the JSON file has the .json
extension, which is common for JSON documents.
Loading Data Using json.load
and json.loads
Python’s json
module provides two methods to read JSON data:
json.load(file)
: Used to read JSON data from a file object.json.loads(string)
: Used to convert a JSON formatted string into a Python object.
- Using
json.load
with a file:
import json
with open('data.json') as f:
data = json.load(f)
print(data) # Outputs a dictionary
- Using
json.loads
with a string:
import json
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string)
print(data) # Outputs a dictionary
These methods decode JSON data into Python dictionaries (dict
) or lists (array
). This deserialization allows you to work with data in your Python programs easily.
Working with JSON Data in Python
JSON data is widely used in web applications for data exchange. It is crucial for developers to read, write, and handle JSON data efficiently. This guide covers essential techniques, helping developers parse JSON into Python objects and manage complex JSON structures.
Parsing JSON to Python Objects
To parse JSON data into Python objects, use Python’s built-in json module. This module provides methods like json.load()
and json.loads()
. json.load()
reads JSON data from a file, turning it into a dictionary or list.
Example:
import json
with open("data_file.json", "r") as file:
data = json.load(file) # Parses JSON file into a dictionary or list
For JSON strings, use json.loads()
to convert the string into a Python object:
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string) # Parses JSON string into a dictionary
These methods handle various data types like str
, int
, float
, True
, False
, and None
. If inputs are not valid, a ValueError
or TypeError
will be raised.
Handling Complex JSON Structures
Complex JSON structures may include nested lists and dictionaries. Parsing these correctly involves navigating through the nested elements.
Example:
json_string = '''
{
"name": "John",
"age": 30,
"children": [
{"name": "Anna", "age": 10},
{"name": "Peter", "age": 5}
]
}
'''
data = json.loads(json_string)
print(data["children"][0]["name"]) # Outputs: Anna
For custom JSON objects, use the object_hook
parameter in json.loads()
. This allows custom parsing logic for JSON data.
Example:
def custom_parser(dct):
if 'name' in dct:
return f"Name: {dct['name']}"
return dct
json_string = '{"name": "Anna", "age": 10}'
data = json.loads(json_string, object_hook=custom_parser)
print(data) # Outputs: Name: Anna
Tips for Effective JSON Handling
1. Pretty Print JSON: Use json.dumps()
with the indent
parameter to make JSON data easier to read.
json_string = '{"name": "John", "age": 30}'
parsed = json.loads(json_string)
print(json.dumps(parsed, indent=4))
2. Sorting Keys: Use the sort_keys
parameter in json.dumps()
to sort keys in the JSON output.
print(json.dumps(parsed, sort_keys=True, indent=4))
3. Managing Whitespaces: Use the separators
parameter to manage spaces in JSON output.
print(json.dumps(parsed, separators=(',', ':')))
4. Error Handling: Handle possible errors like ValueError
when parsing JSON to prevent crashes.
try:
json.loads('{"name": "John", "age": "thirty"}')
except ValueError as e:
print("Invalid JSON:", e)
These tips ensure efficient and error-free handling of JSON data in Python.
Frequently Asked Questions
This section answers common questions on how to work with JSON files in Python. It covers loading JSON data into dictionaries, using Pandas, and handling multiple files.
How can I load a JSON file into a dictionary in Python?
To load a JSON file into a dictionary, use Python’s built-in json
module. Open the file with open()
, and then use the json.load()
method. This will convert the JSON data into a Python dictionary.
What is the method to read a JSON file using Pandas in Python?
Pandas can read JSON files using the read_json()
method. You need to import pandas first. This method takes the file path as an argument and returns a DataFrame. It is useful for data analysis tasks.
How can one iterate over a directory to read multiple JSON files in Python?
To read multiple JSON files in a directory, use the os
module to list all files in the directory. Loop through the list and open each file using the json
module. You can then load the data into a dictionary or other data structure.
What is the correct way to write data into a JSON file in Python?
To write data into a JSON file, open the file in write mode using open()
. Then use the json.dump()
method to write the data. This method serializes the data and saves it in the specified file.
How to read a JSON file from a different directory in Python?
To read a JSON file from a different directory, provide the full path to the open()
method. If the path includes backslashes (Windows), use raw string notation or double backslashes to avoid issues.
What approach should be taken to parse a list from a JSON file in Python?
To parse a list from a JSON file, load the file using json.load()
. Check if the data type of the loaded data is a list. If it is a list, you can directly iterate over it or access its elements.