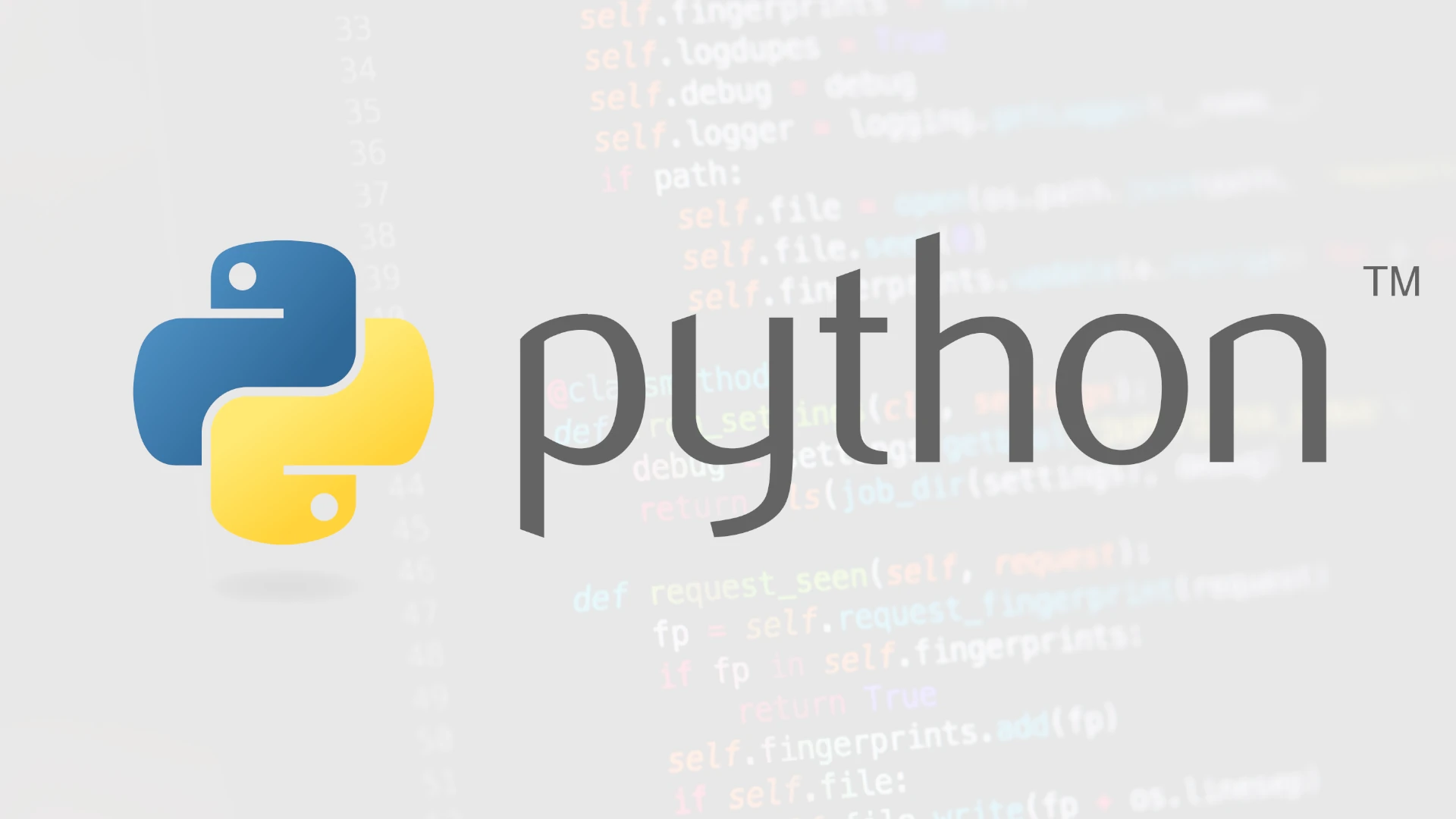
Adding a new column to a Pandas DataFrame is essential for many data analysis tasks. To insert a new column, you can use methods such as insert(), assign(), and loc[]. These functions allow you to efficiently modify and expand your data structures.
For instance, using the insert() method, you can specify the location and the value of the new column. Alternatively, assign() is useful for adding multiple columns simultaneously. Both approaches offer different flexibilities based on your specific needs.
Modifying a DataFrame doesn’t stop there. You can also create new columns by performing operations on existing ones. Methods like apply() combined with mathematical operations can help automate these processes, making data manipulation smoother and more effective.
Enhancing Your Pandas DataFrames
Adding New Columns
Pandas makes it easy to add new columns to your DataFrame. You can add columns with simple assignment, using the .insert()
method for precise positioning, the .assign()
method for creating columns based on existing data, or by concatenating with another DataFrame or Series.
Simple Assignment
The most straightforward method is direct assignment using square brackets []
. Let’s say you have a DataFrame df
and want to add a new column called Age
.
df['Age'] = [25, 30, 35, 40]
Inserting Columns at Specific Positions
To add a column at a specific position, use the .insert()
method.
df.insert(2, "City", ["New York", "London", "Paris", "Tokyo"])
This will insert the City
column at index 2 (the third column).
Assigning Based on Existing Data
The .assign()
method is useful for creating new columns based on existing data within the DataFrame.
df = df.assign(IsAdult = df['Age'] >= 18)
This will create a new column IsAdult
with boolean values based on the Age
column.
Concatenating DataFrames or Series
You can also add columns by concatenating with another DataFrame or Series that shares the same index as the original DataFrame.
import pandas as pd
new_data = pd.Series([100, 200, 300, 400], name='Score')
df = pd.concat([df, new_data], axis=1)
This will add the Score
column to the DataFrame df
.
Comparison of Methods
Method | Description | Example |
---|---|---|
Simple Assignment | Assign values directly to a new column. | df['NewColumn'] = values |
.insert() | Insert a column at a specified index. | df.insert(2, "NewColumn", values) |
.assign() | Create a new column based on existing data. | df = df.assign(NewColumn=df['ExistingColumn'] * 2) |
pd.concat() | Concatenate a DataFrame or Series as a new column. | df = pd.concat([df, new_data], axis=1) |
Key Takeaways
- Use insert(), assign(), and loc[] to add columns in Pandas
- Apply mathematical operations to create new columns
- Different methods offer flexibility for data manipulation
Understanding DataFrames in Pandas
Pandas DataFrames are essential tools in Python for handling and analyzing tabular data. This section explores their structure and how to manipulate them effectively.
DataFrame Structure
A DataFrame in pandas is a two-dimensional labeled data structure, similar to a table in a relational database. It consists of rows and columns, where each column can have different data types such as integers, floats, or strings.
- Rows and Columns: Data is stored in rows and columns, providing a clear and organized way to manage the dataset.
- Index: Each row has an index label, helping to access and manipulate data efficiently.
- Columns: Column names serve as labels for the data they hold, ensuring that complex datasets remain understandable.
An example of a DataFrame creation:
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]}
df = pd.DataFrame(data)
print(df)
This code snippet shows a simple DataFrame with names and ages.
Data Manipulation Basics
Manipulating a DataFrame is straightforward with the pandas library. Basic operations include adding, deleting, and modifying columns or rows.
Adding Columns: You can add a new column to a DataFrame using various methods. One simple way is to assign a list to the new column.
df['City'] = ['New York', 'Los Angeles']
Deleting Columns: To remove a column, use the drop
method:
df = df.drop('City', axis=1)
Updating Data: Modifying values in a DataFrame can be done by direct assignment. For example, to update the age of the first entry:
df.at[0, 'Age'] = 26
These basic operations are pivotal in preparing data for analysis or machine learning tasks. Understanding how to efficiently manage DataFrames boosts productivity and ensures data remains clean and well-organized.
Adding Columns to DataFrames
When working with pandas DataFrames, adding new columns is a common task. This can be done in multiple ways, each offering different flexibility and use cases.
Using Assign Method
The assign()
method can be used to add a new column to an existing DataFrame. This method allows you to create new columns by passing new column names as keyword arguments and their respective values.
df = df.assign(new_column=df['existing_column'] + 10)
This statement will add a column named new_column
to DataFrame df
, with each entry being the result of the calculation existing_column + 10
. It retains the original DataFrame, returning a new one if the inplace parameter is left as default.
Using Insert Method
The df.insert()
method adds a column at a specified position. It takes four parameters: the index position, the name of the column, the data to be added, and whether to allow duplicates.
df.insert(loc=2, column='new_column', value=[1, 2, 3], allow_duplicates=False)
This will insert new_column
at the third position in the DataFrame df
. Attempting to insert a column with the same name will raise a ValueError
unless allow_duplicates
is set to True.
Direct Assignment
Direct assignment is straightforward and is often the fastest way to add a new column. You simply use the new column name within brackets and assign it a Series, list, or constant value.
df['new_column'] = [1, 2, 3]
This method automatically places the new column at the end of the DataFrame. It’s efficient and easy to use for quick additions.
Adding Multiple Columns
Adding multiple columns can be done through a combination of methods. You can use assign()
for multiple columns or direct assignment for each column. Using dictionaries with assign()
is another smart approach.
df = df.assign(new_col1=[1, 2, 3], new_col2=[4, 5, 6])
Using dictionaries allows you to group all new columns together, keeping your code clean and organized. You can also add columns conditionally using apply()
with lambda functions.
By understanding these methods, you can effectively add new columns to pandas DataFrames, making your data manipulation tasks easier and more efficient.
Frequently Asked Questions
This section addresses common queries about adding columns to a Pandas DataFrame. It covers creating empty columns, adding default values, inserting columns at specific indices, deriving columns from existing columns, and other related tasks.
How can I create an empty column in a DataFrame in Pandas?
To create an empty column in a DataFrame, you simply assign None
or NaN
values to a new column name. Here’s an example:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3]})
df['New_Column'] = None
What is the syntax for adding a new column with default values to a Pandas DataFrame?
To add a new column with default values, you assign a constant value to the new column. For instance, to add a column filled with zeros:
df['New_Column'] = 0
How can one insert a column at a specific DataFrame index using Pandas?
To insert a column at a specific index, you use the insert()
method. This method takes the index, column name, and values as arguments:
df.insert(1, 'New_Column', [10, 20, 30])
What is the method to derive a new column in a DataFrame based on the values of other columns?
You can derive a new column based on existing columns by applying a function or using an expression:
df['New_Column'] = df['A'] * df['B']
How do you add a column populated from a Python array to a DataFrame using Pandas?
To add a column from a Python array, assign the array to the new column. Ensure the length of the array matches the DataFrame’s row count:
new_data = [7, 8, 9]
df['New_Column'] = new_data
What are the steps to append a column to the end of a Pandas DataFrame?
To append a column to the end, assign the values directly to a new column name:
df['New_Column'] = [5, 6, 7]
This method appends the new column to the end of the DataFrame by default.