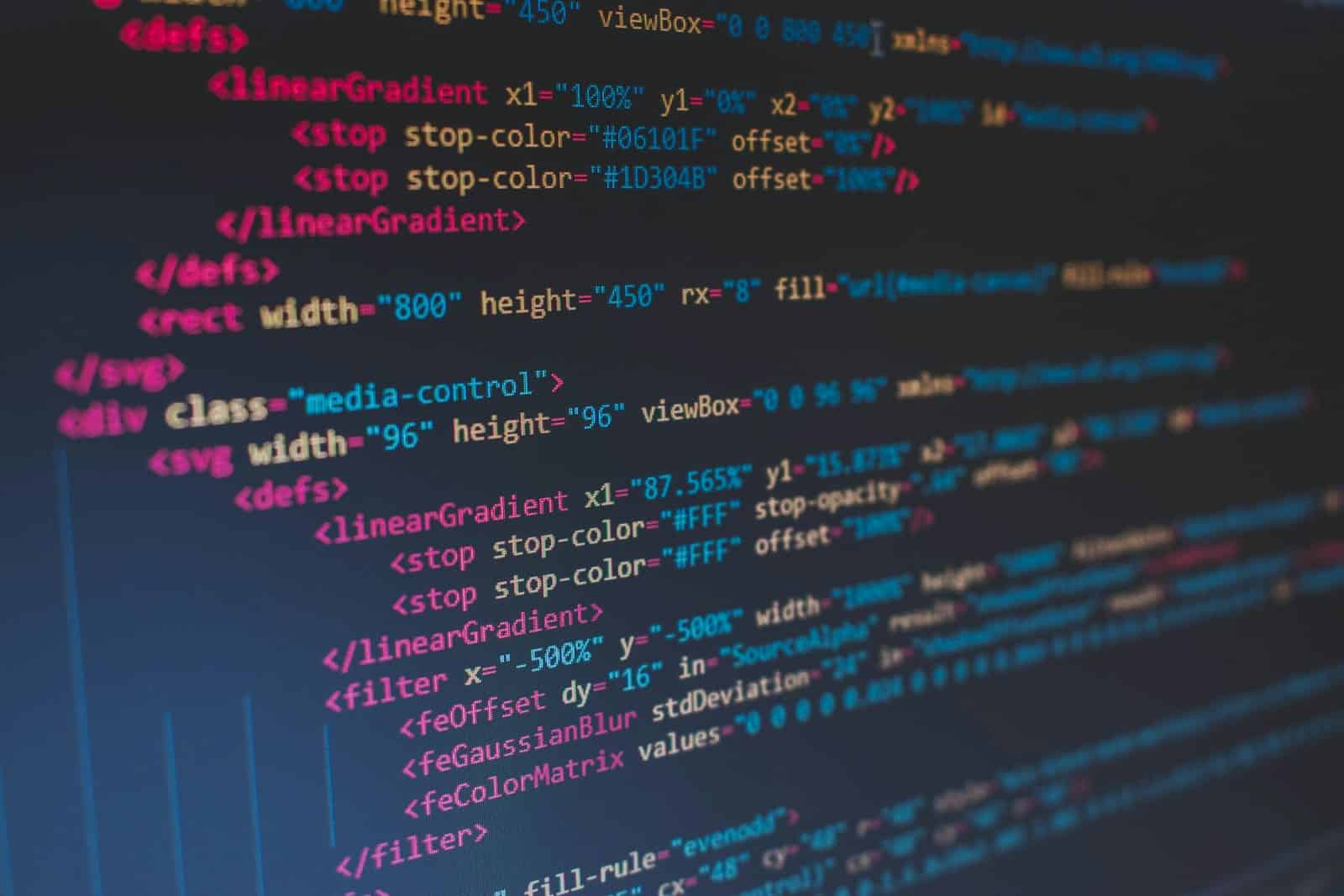
Quicksort is a powerful and efficient sorting algorithm that uses a divide-and-conquer approach. Developed by Tony Hoare in 1959, it remains widely used due to its quick performance, especially with large datasets. Quicksort works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays based on whether they are less than or greater than the pivot.
The simplicity of Quicksort lies in its recursive nature, which allows it to efficiently sort both small and large collections of data. For those learning about algorithms, understanding Quicksort provides valuable insight into the mechanics of sorting and how divide-and-conquer techniques can optimize performance. Implementations of Quicksort can be found in various programming languages, including Python, Java, and C++.
Besides its theoretical importance, Quicksort’s practical applications make it an essential tool for developers. It is often used in software development and data processing due to its reliability and speed. Learning how to implement and optimize Quicksort can help improve your programming skills and understanding of efficient data handling.
Understanding Quicksort
How Quicksort Works
Quicksort is a divide-and-conquer algorithm. It works by picking an element from the array, called the pivot. The array is then rearranged to put all elements smaller than the pivot before it and all elements larger than the pivot after it. This process is called partitioning.
After partitioning, the pivot is in its final sorted position. Quicksort then recursively sorts the subarray to the left of the pivot and the subarray to the right of the pivot. This process continues until the entire array is sorted.
Key Steps
- Choose a pivot: The pivot can be chosen in several ways, such as the first element, the last element, or a randomly selected element.
- Partition: Rearrange the array so that elements smaller than the pivot are before it and elements greater than the pivot are after it.
- Recursive Sorting: Recursively apply quicksort to the subarray of smaller elements and the subarray of larger elements.
Why Quicksort is Efficient
Quicksort has an average time complexity of O(n log n), which is considered efficient for large datasets. The algorithm’s efficiency comes from its divide-and-conquer approach, which breaks down the problem into smaller, more manageable subproblems.
Worst-Case Scenario
In the worst-case scenario, where the chosen pivot is consistently the smallest or largest element, quicksort can have a time complexity of O(n^2). This can be mitigated by choosing a random pivot or using a different sorting algorithm for small subarrays.
Comparison with Other Sorting Algorithms
Algorithm | Average Time Complexity | Worst-Case Time Complexity | Space Complexity |
---|---|---|---|
Quicksort | O(n log n) | O(n^2) | O(log n) |
Merge Sort | O(n log n) | O(n log n) | O(n) |
Heap Sort | O(n log n) | O(n log n) | O(1) |
Insertion Sort | O(n^2) | O(n^2) | O(1) |
When to Use Quicksort
Quicksort is a good choice when you need to sort a large dataset quickly and don’t mind the possibility of a worst-case scenario. It is commonly used in programming libraries and real-world applications due to its efficiency and ease of implementation.
Key Takeaways
- Quicksort is an efficient sorting algorithm using divide-and-conquer.
- The pivot element is key to partitioning the array.
- Understanding Quicksort enhances programming and data handling skills.
Quicksort Fundamentals
Quicksort is a popular sorting algorithm that uses the divide and conquer method to sort arrays. Key processes include partitioning the array and selecting a pivot element.
Algorithm Overview
Quicksort begins by selecting a pivot element from the array. The pivot can be chosen in various ways, like always picking the first element, the last element, or a random element.
Next, the array is partitioned so that elements less than the pivot are on one side, and elements greater than the pivot are on the other side. The pivot moves to its correct position in the sorted array.
After partitioning, the algorithm recursively sorts the subarrays on either side of the pivot. This continues until the base case is reached, which is a subarray of zero or one element. Quicksort is efficient, performing well on average, but it can be slower in the worst-case scenario.
Partitioning Strategies
The partitioning step is crucial for Quicksort’s efficiency. In the Lomuto partition scheme, the pivot is moved to the end of the array, and a “failsafe” index is used to place elements less than the pivot to the beginning of the array.
In the Hoare partition scheme, two indices move towards each other from opposite ends of the array. When they find elements on the wrong side of the pivot, they swap them.
Choosing the right partition method and pivot selection strategy can impact the performance of Quicksort, particularly how balanced the subarrays are, which directly affects the time complexity of the sort. Efficient partitioning allows Quicksort to sort quickly and effectively.
For more detailed information on the partitioning process, you can read CSEStack’s detailed tutorial and GeeksforGeeks’s explanation.
Implementation and Optimization
Implementation and optimization of Quicksort involve various techniques and considerations specific to each programming language, as well as comparisons to other sorting algorithms. Important points include language-specific implementations, space complexity in different scenarios, and improvements like dual-pivot Quicksort.
Language-Specific Implementations
Quicksort can be implemented in many programming languages, each offering unique tools and libraries.
Java: In Java, Quicksort is often implemented using recursion. Java’s standard library provides an optimized version, utilizing dual-pivot Quicksort which minimizes the number of comparisons.
Python: Python’s implementation is straightforward. The standard sort()
function utilizes Timsort, which merges Quicksort and merge sort.
C++: C++ offers flexibility with its standard library sort()
function which uses a variant of Quicksort. Performance can be tuned further by leveraging manual memory management.
Optimizing Quicksort
Optimization of Quicksort focuses on reducing time and space complexities.
Pivot Selection: Choosing an effective pivot is crucial. A common method uses the median of medians to avoid worst-case scenarios.
Tail Call Optimization: Reducing stack usage is important. Tail call optimization helps minimize space complexity to O(log n).
Randomized Algorithms: Randomizing the pivot ensures better performance on average, improving resilience against worst-case inputs. This reduces the chances of consistently picking poor pivots.
Comparison to Other Sorts
Quicksort’s performance varies when compared to other sorting algorithms.
Merge Sort: Both have O(n log n) average time complexity. Merge sort is stable but uses more space compared to Quicksort.
Insertion Sort: Quicksort generally outperforms insertion sort, especially for large datasets. Insertion sort can be faster for nearly sorted arrays.
Selection Sort: Quicksort is superior in terms of both time and space complexity. Selection sort is not practical for large datasets due to its O(n^2) time complexity.
Using these techniques ensures Quicksort remains a powerful and efficient sorting method, suitable for many applications.
Frequently Asked Questions
Quicksort is a highly efficient sorting algorithm that uses a divide-and-conquer strategy. It is known for its performance and simplicity.
How is the time complexity of Quicksort calculated?
Quicksort’s average time complexity is O(n log n). This is calculated based on its divide-and-conquer approach where the array is divided into smaller sub-arrays and recombined. The worst-case time complexity is O(n²) when the pivot selection is poor, but this is rare with good pivot choices.
What are the steps involved in implementing Quicksort in Java?
Implementing Quicksort in Java involves selecting a pivot element, partitioning the array around the pivot, and recursively sorting the sub-arrays. Detailed implementation steps can be found here.
In what scenarios is Quicksort considered less efficient than other sorting algorithms?
Quicksort may be less efficient when the data is already sorted or nearly sorted, leading to the worst-case time complexity of O(n²). For such cases, algorithms like Merge Sort or Heap Sort are often preferred.
Can you provide an example of Quicksort implementation in Python?
A simple Quicksort implementation in Python starts by choosing a pivot and partitioning the list. Here’s an example:
def quicksort(array):
if len(array) <= 1:
return array
pivot = array[len(array) // 2]
left = [x for x in array if x < pivot]
middle = [x for x in array if x == pivot]
right = [x for x in array if x > pivot]
return quicksort(left) + middle + quicksort(right)
print(quicksort([3,6,8,10,1,2,1]))
How does Quicksort differ in performance when implemented in C versus C++?
In C, Quicksort can be implemented with raw pointers, giving more fine-grained control over memory and potentially better performance. In C++, templates and STL (Standard Template Library) can be used to implement Quicksort more flexibly. Both implementations follow the same basic principles but may differ in execution speed and ease of use.
What are best practices for visualizing the Quicksort algorithm during operation?
To visualize Quicksort, use color-coded bars to represent array elements and highlight the pivot and partitions during the sort. Tools like visualgo.net or writing custom visualization code in Python with libraries like matplotlib can help. Visualization helps in understanding the partitioning and recursive sorting steps efficiently.