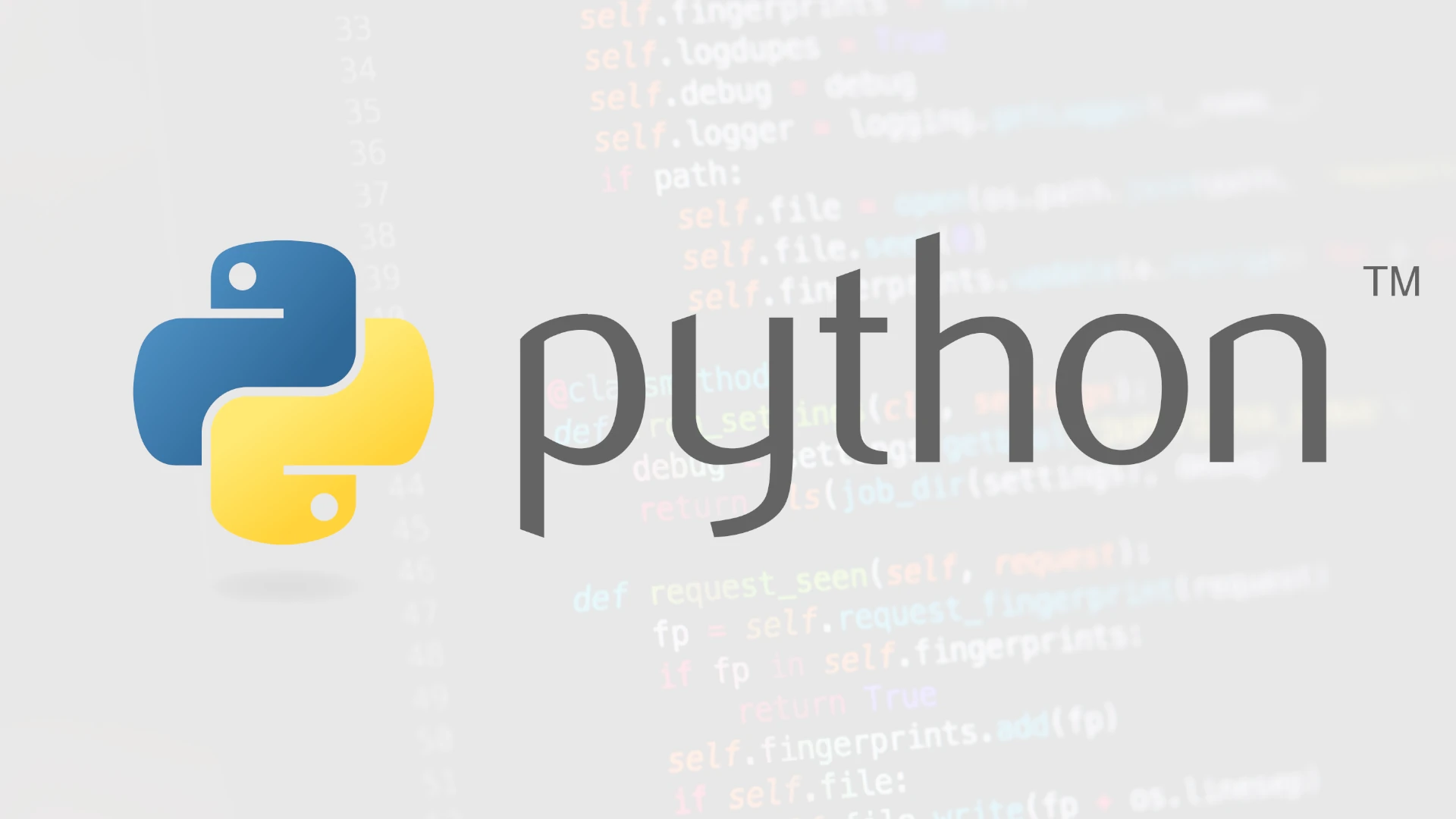
Incrementing a variable is a fundamental concept in Python. It involves increasing the value of the variable, usually by adding one to the current value. Unlike some other programming languages like C++ or Java, Python does not have a specific increment operator, but it offers a simple alternative method.
Python: Incrementing by 1
Method | Expression | Example | Output |
---|---|---|---|
Assignment Operator (+= ) | variable += 1 | x = 5 x += 1 | x becomes 6 |
Addition Operator (+ ) | variable = variable + 1 | x = 5 x = x + 1 | x becomes 6 |
Increment Operator (pre-increment) | ++variable (not available in Python) | Not applicable | |
Increment Operator (post-increment) | variable++ (not available in Python) | Not applicable | |
Function (operator.add(variable, 1) ) | operator.add(variable, 1) | x = 5 x = operator.add(x, 1) | x becomes 6 |
Notes:
- Python does not have built-in increment or decrement operators (
++
and--
). - The most common way to increment a variable by 1 is using the assignment operator (
+=
). - The addition operator (
+
) can also be used, but it creates a new object and reassigns it to the variable. - Functions like
operator.add
offer alternative ways, but are less commonly used.
Understanding Incrementation in Python
The Augmented Assignment Operator (+=
) is typically used for incrementing a variable’s value. Here’s the syntax broken down:
variable += value
The variable is what you’re looking to increase, and the value is how much you wish to add – usually one for direct increment.
For example:
counter = 0
counter += 1
In this case, counter
is the variable, and we’re adding 1 to it. The left operand (counter
) is increased by the value on the right (1), and the result is stored back in counter
.
Though Python lacks an explicit increment operator, +=
serves as both an assignment and an increment operator. To understand this, you must grasp that an operand is an object manipulated by an operator, and in x += 1
, x
is the operand.
Remember, while Python is different from some languages, its use of the +=
operator for incrementation is quite effective and simple to understand even when you’re just starting out with programming.
Now, let’s increment x
by 1:
x = 5
x += 1 # x is now 6
Here, the variable x
with an initial value of 5 is increased by 1. Following the operation, x
holds the new value, 6. This completes the incrementation process in Python using the augmented assignment operator.
Utilizing the += Operator
When working with numbers in Python, the +=
operator offers a quick and efficient way to add to the value of a variable. This addition shortcut is not just about saving keystrokes; it makes the code cleaner and more readable.
Syntax of += Operator
The +=
operator, also known as the addition assignment or augmented assignment operator, simplifies adding a number to a variable with elegant syntax. The basic form looks like this:
variable += value
This line of code translates to taking the current value of variable
, adding value
to it, and then updating variable
with the new sum. The +=
operator is part of a family of similar augmented assignment operators, each corresponding to a basic arithmetic operation.
Examples Using += Operator
To see the +=
operator in action, let’s start with a simple scenario:
score = 0
score += 1
In this example, the number 1
is added to score
. If score
was zero, it now holds the value 1
. For multiple additions, the +=
operator can be used repeatedly:
counter = 10
counter += 1 # counter is now 11
counter += 1 # counter is now 12
In both examples, the +=
operator serves to add 1
to the variable on the left efficiently. It’s a blend of addition and assignment that modifies the original variable in one straightforward expression.
Common Increment Operations
When talking about incrementing in Python, it generally means to increase the value of a variable. In Python, there isn’t a specific increment or decrement operator, but common tasks like these are still simple and straightforward.
Incrementing Integers
To increment an integer in Python, you typically add one to the variable. For example:
counter = 0
counter += 1
This is known as the augmented assignment operator and it updates the value of counter
to 1.
Adding Strings and Concatenation
When it comes to strings, “incrementing” usually refers to appending or adding onto the string. Concatenation is the process of joining strings together:
greeting = "Hello, "
greeting += "World!"
Now, greeting
will be "Hello, World!"
as the second string is added to the first.
Working with Lists
For lists in Python, incrementing could mean appending elements or combining lists. Here’s how:
# Appending an item
my_list = [1, 2, 3]
my_list.append(4)
# Combining two lists
another_list = [5, 6]
my_list += another_list
After these operations, my_list
will contain [1, 2, 3, 4, 5, 6]
. The append()
method adds an individual element while the +=
operator combines two lists.
Control Structures and Incrementing
When programming in Python, control structures such as loops are fundamental in managing the flow of your code. They are key when you need to increment a value through each iteration. Let’s look at how to use incrementing effectively in both for
and while
loops.
Incrementing in a For Loop
In a for
loop, iteration happens over a sequence. To increment a counter by 1 in each iteration, you can use the range()
function.
for counter in range(start, stop):
# Your code here
Here’s a simple example:
Example:
for i in range(1, 6):
print(i)
This loop will print numbers from 1 to 5. The range()
function generates a sequence of numbers, and the for
loop executes its code block once for each number in that sequence.
Using Increment in While Loops
A while
loop runs as long as a condition is True
. To increment within a while
loop, you typically initialize a counter before the loop, then increase it within the loop’s body.
Initialization:
count = 0
While Loop:
while count < 5:
count += 1
print(count)
This loop continues running and incrementing count
until count
is equal to 5. Each iteration increases count
by 1 using the +=
operator, which is the standard way to increment a value in Python.
Advanced Incrementing Concepts
Understanding how to increment variables by 1 in Python involves more than the basic +=
operator. This section delves into some of the nuanced ways of managing variable incrementation.
Pre-Increment and Post-Increment
Python does not support the pre-increment (++i
) and post-increment (i++
) operators found in some other programming languages. The functionality they represent, however, can still be achieved. In Python, variable incrementation, like i = i + 1
, is always executed before the result is used in any further expressions, effectively making it a pre-increment operation.
The Concept of Immutability
Immutability in Python refers to the characteristic of some objects to be resistant to change. For example, numbers are immutable. When you think you are modifying an integer’s value, what you’re actually doing is creating a new integer object and assigning it to the same variable. This means each increment operation is not changing the original value but is creating a new incremented one. This concept is crucial when dealing with incrementation within functions and understanding Python’s behavior with variable assignment and modification.
Comparative Incrementing in Other Languages
When coding, increasing a variable’s value by one is a common action. Different programming languages have their own ways of doing this.
Incrementing in C++ and JavaScript
In languages like C++ and JavaScript, incrementing a number by one is typically done using the ++
operator. This operator is succinct and incorporated into the very fabric of these programming languages.
C++:
int x = 5;
x++; // x now becomes 6
In C++, x++
is an example of the ++
operator being used in its postfix form. The value of x
is increased by one after its current value has been used. Conversely, ++x
is the prefix form, where x
is incremented before it’s used.
JavaScript:
let y = 5;
y++; // y is now 6
Similarly, in JavaScript, the ++
operator functions in the same way, allowing for both prefix and postfix increments. It’s a go-to technique for concise and straightforward incrementation in code.
Both C++ and JavaScript also offer a --
decrement operator, which works like the increment operator but decreases the value by one.
Python, on the other hand, does not have the ++
operator. To increment a value by one in Python, you would simply use +=
like so:
Python:
x = 5
x += 1 // x is now 6
Understanding these differences is important for programmers who transition between languages, as each language has its own syntax and rules.
Error Handling and Best Practices
When writing Python code, especially dealing with incrementing values, one should pay close attention to error handling to ensure the program runs smoothly and is easy to read. Let’s look at common practices for dealing with increments and how to handle potential errors effectively.
Handling TypeError in Increment Operations
If someone mistakenly tries to increment a non-numeric type, Python will raise a TypeError
. It is crucial to catch and handle this to prevent a program from crashing. For example, when performing an increment operation:
try:
var += 1
except TypeError:
print("Variable is not a number")
This simple structure ensures that the program informs the user of the mistake without halting execution unexpectedly.
Pythonic Alternatives to Incrementing
In Python, the most common method to increment a variable is by using the +=
syntax, which is both concise and clear. For readability and maintaining a Pythonic style, one might use comprehension or generator expressions for bulk increments. For instance:
numbers = [1, 2, 3]
numbers = [x + 1 for x in numbers]
This line replaces a traditional loop with a single, Pythonic line that is easy to understand and efficient.
By sticking to these practices, one can write code that is both robust against errors and aligns with Python’s emphasis on readability and simplicity.
Frequently Asked Questions
Incrementing values is a common operation in Python programming. This section answers some popular questions on how to increase numbers effectively within different Python data structures and scenarios.
How can you add 1 to an integer in a Python for loop?
In a for loop, you simply include the increment operation within the loop’s body. You can enhance an integer’s value by using the +=
operator like so: number += 1
.
What is the correct syntax to increase the value of a variable by 1?
To bump up a variable’s value by 1, you would use the +=
operator, which is concise and commonly used: variable += 1
.
Can you automatically increment a number in each iteration of a Python while loop?
Yes, within a while loop, you can automatically escalate a number each time the loop cycles through. It’s done by placing the incrementing statement number += 1
inside the loop.
How do you enhance a numerical value in a string by 1?
You must first convert the numerical part of the string to an integer, increment it, and then convert it back to a string. For example, str(int('42') + 1)
would update the string from ’42’ to ’43’.
What’s the best practice for incrementing the elements of a list in Python?
The optimal approach for increasing each element in a list by 1 is to use a list comprehension: [x + 1 for x in original_list]
.
In a Python dataframe, how can you increment a particular column’s values by 1?
To augment values of a dataframe column, use the +=
operator on the column: dataframe['column_name'] += 1
. This will increase each value in the column by 1.